Background - Repetitive Processes w/ Git
You may or may not find this script particularly useful--this post is really about the power of bash scripts to help manage workflow. I do, however, find myself checking out new Git branches somewhat frequently. So, with the extra time in my schedule, I decided to have a little fun and see if I could automate some of that Git workflow with a simple script. Checking out a branch with git isn't a particularly complicated process: git checkout -b <branchname>. I could shorten this with a Bash Alias if it really bothered me--although, it's not that long or complicated, all things considered. I do, however, often find myself on one branch, needing to checkout a new branch from Master. When that's the case, my keystrokes inflate rather quickly. I need to find my way to master, almost always need to pull so I can be sure Master is up to date, and then checkout my new branch. That's three commands that I could greatly simplify with a more or less simple script. So, that's where I'm coming from today. I want a script that can handle checking out a branch from my current branch, or from Master if need be.
The Script
Cutting to the chase, this is what it looks like. I'll explain the process below:
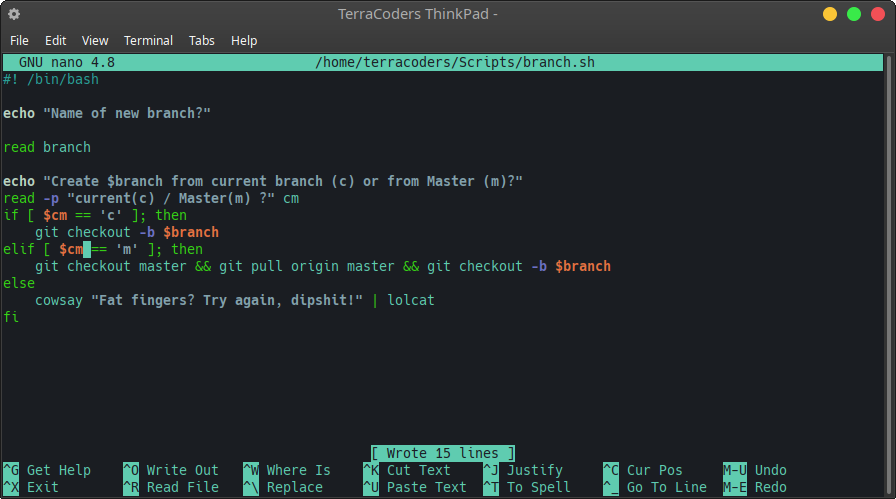
Step 1 - Prepping a Scripts Folder
If you don't have a /Scripts folder setup, that's where you'll want to start. To keep things simple, I put mine in my user folder (i.e., /home/username/Scripts. You'll want to be sure this folder gets added to your $PATH. You can do that on the command line with the following:
$ export PATH=$PATH:/home/username/Scripts
// TO VERIFY IT WORKED
$ echo $PATH
Once your directory is setup, you can start working on your script. I prefer Nano, but with whatever editor you like, create a file named branch.sh in the /Scripts directory.
Step 2 - Hashbang #!
The first line in your file basically describes how the file will be executed. In our case, we want to use the Bash shell, so we start with:
#! /bin/bash
Step 3 - Name for a New Branch
Since we'll use this script to create a new Git branch, we'll probably want to start by asking our user for a name. We can store that name as a variable and then pass it to some logic determining whether we want to create the branch from whatever branch we're currently on or from Master. We'll use echo to ask for the new branch name, and then read to capture it as a variable--like so:
echo "Name of new branch?"
read branch
# WHATEVER THE USER ENTERS AT THE read PROMPT WILL AUTOMATICALLY GET STORED IN A VARIABLE CALLED $banch
NOTE: optionally, you could include a git status before echoing, "Name of new branch?"--this would allow you to see which branch you're currently on. I don't need this for my Xubuntu machine since I can see the branch status in the command prompt whenever I'm in a repository. Still, it could definitely be useful.
Step 4 - Multiple Choice Logic
Here's where things get fun. We want to offer two choices: when prompted, the user can either create this new branch from the current branch (by typing 'c'), or create it from Master (by typing 'm'). Just like above, we can use read again to prompt them for the choice. Depending on the answer, we can put together an if statement to determine a path forward. Here's what that will look like:
# WE CAN REFERENCE THE $banch VARIABLE FROM STEP 3 IN OUR QUESTION HERE
echo "Create $branch from current branch (c) or from Master (m)"
# -p OPTION PUTS THE PROMPT IN LINE WITH THE COPY; cm BECOMES A NEW VARIABLE ($cm) STORING THE ANSWER
read -p "current (c) / Master (m)?" cm
# THE USER SHOULD EITHER ENTER 'c' OR 'm'; THAT VALUE WILL BE STORED IN $cm
# AND NOW THE if LOGIC
if [ $cm == 'c' ]; then
# CHECKOUT FROM CURRENT, SO...
git checkout -b $branch
# ELSE IF...
elif [ $cm == 'm' ]; then
# CHECKOUT FROM MASTER, SO...
git checkout master && git pull origin master && git checkout -b $branch
# DEFINITELY SAVED A FEW KEYSTROKES THERE! NOW THE FUN PART...
else
# IF $cm HAS ANY VALUE OTHER THAN 'c' OR 'm'...
cowsay "Fat fingers? Try again, Dipshit!" | lolcat
# YOU COULD JUST echo THIS, BUT cowsay AND lolcat ARE THE BEST!
fi
Hopefully most of that is pretty straightforward. The syntax here isn't too different from PHP or JavaScript. Just be aware that for that else statement (i.e., what to do when a user doesn't choose 'c' or 'm'), you'll need to have both cowsay (sudo apt-get install cowsay) and lolcat (sudo apt-get install lolcat) installed. Save your script and let's get to the final step.
Step 5 - Calling the Script
You should now be able to call the script by simply typing it's path. You'll, of course, need to be within one of your Git projects:
/path/to/git_repository $ ~/Scripts/branch.sh
A much easier way of doing this, however, is to simply add the script to your .bash_aliases file.
alias branch='~/Scripts/branch.sh'
# BE SURE TO RUN source .bashrc AFTER YOU SAVE
Once that's done, you should be good to go. Let's give it a test and see how it runs!
Here's what it looks like when I call the script and choose to create the branch from my current branch:
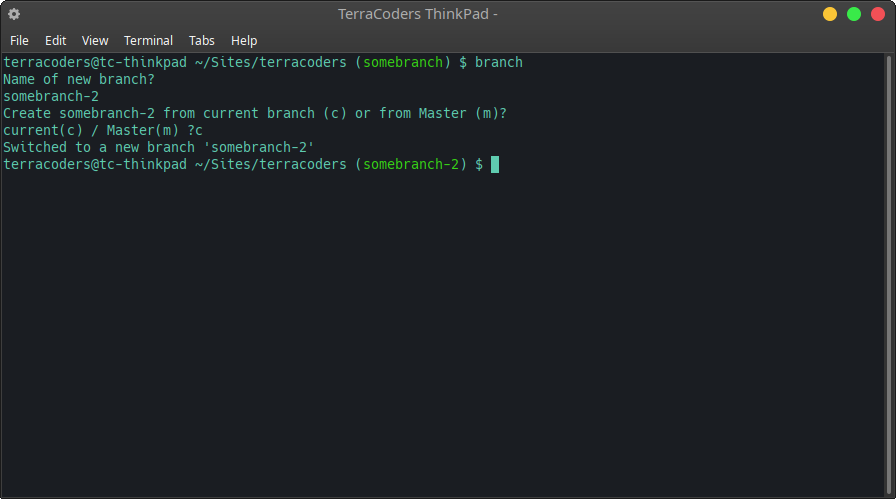
Now let's have a look at the script when checking out the new branch from Master. This method will also pull from remote before checking out the new branch:
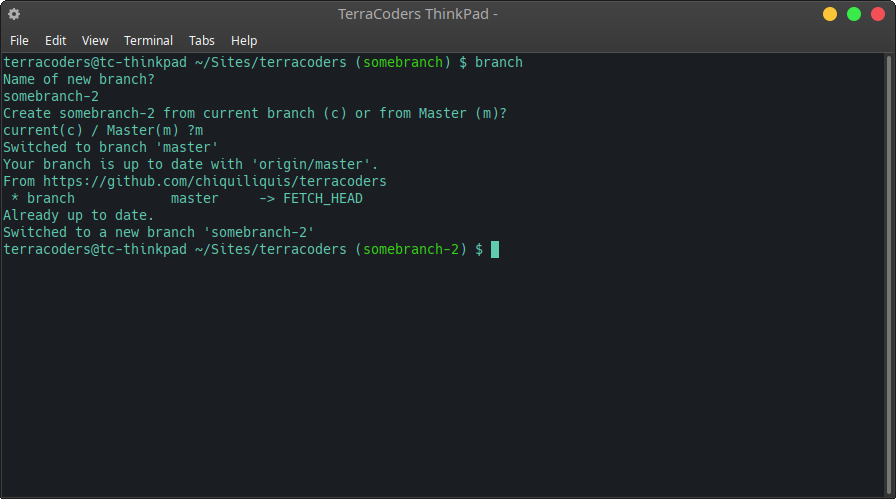
And, last but not least, what happens if we screw up? What happens when we try an option other than 'c' or 'm'?
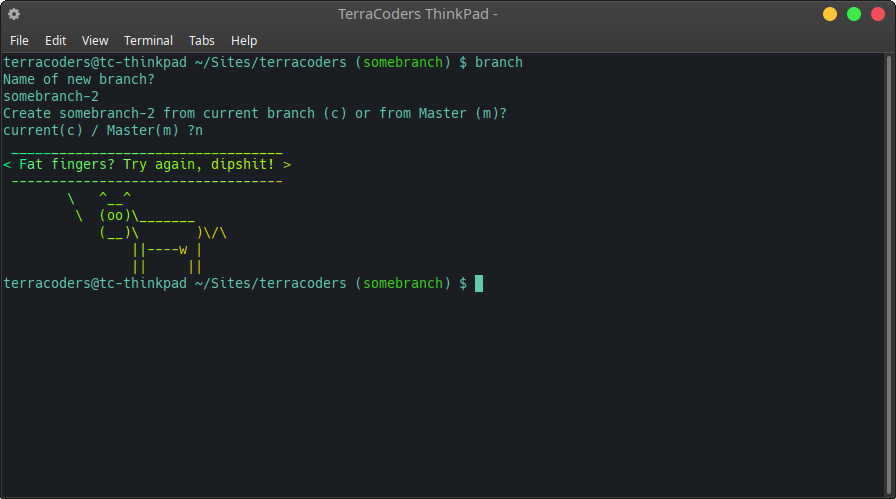
And there you have it! This may not be the most useful script for everyone--but, again, that's not really the point. If there's one point, it's that Bash Scripts can be extremely useful for managing workflow, whatever your particular use-case happens to be. Personally, I just think it's cool you can add a touch of personalization to all those boring hours we developers spend on our terminals!